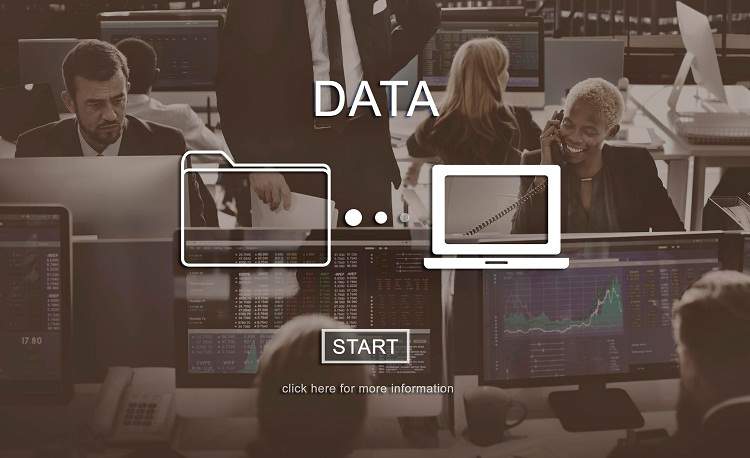
Practical Guide To Angular: Data Binding
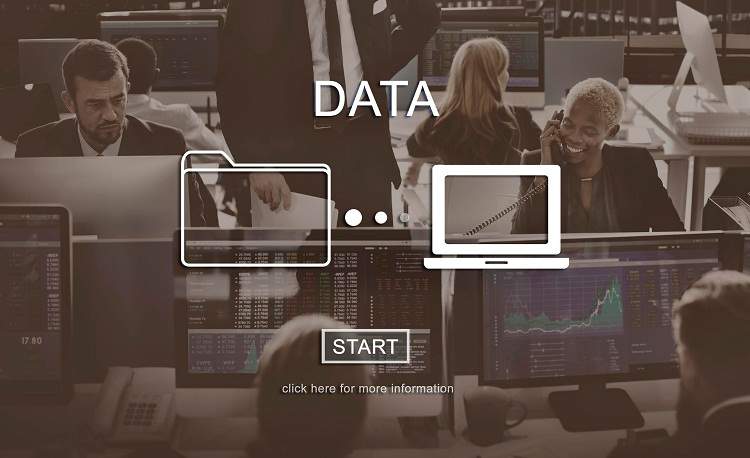
Data Binding is an angular feature that allows information to flow from one layer to another. It can be either unidirectional or bi-directional. Unlike other web development frameworks, Angular framework comes with a set of robust and reliable features and Angular data binding supports both types of binding. It can help developers create dynamic and interactive web apps. Therefore you must learn the practical implementation of this Angular feature. So, without any further ado, let’s get started.
One-way Data Binding
Any changes that you make in the Component while using the one-way-data binding technique, then those changes won’t be reflected in the View or vice versa. This means that in this feature, you only can bind the data either from the Component to the DOM or from DOM to the component.
From Component to View
There are a lot of techniques that you can use to bind the data from component to View. They are as mentioned below:
- Interpolation Binding: The binding expression in the marked-up languages is referred to as Interpolation.
- Property Binding: It is used to set the property of the View elements and a value for the template expression.
- Attribute Binding: It is used to set an attribute property for the View element.
- Class Binding: It is to set the class property for the View element.
- Style Binding: it is to set the style for a View element.
Let us take an example, where an interpolation technique is used to bind two values namely firstName and lastName with the View. They will be enclosed using double curly brackets. and here we will make the exchange of data from the Component to the View. So, if any changes are made in the Component, they will not be reflected in the View and vice versa.
File Name: app.component.ts
1import { Component } from “@angular/core”;
2@Component({
3 selector: ‘app-example’,
4 template: `
5 <div>
6 <strong>{{firstName}}</strong>
7 <strong>{{lastName}}</strong>
8 </div>
9 `
10})
11
12export class AppComponent {
13 firstName: string = “Yallaling”;
14 lastName:string = “Goudar”;
15}
For the next example, we will be trying out property binding techniques where we will try to bind the value namely firstName with the innerHTML property of the span tag. The goal here is to bind the value with an element.
1import { Component } from “@angular/core”;
2@Component({
3 selector: ‘app-example’,
4 template: `
5 <div>
6 <span [innerHTML]=’firstName’></span>
7 </div>
8 `
9})
10export class AppComponent {
11 firstName: string = “Yallaling”;
12}
Now, let’s take an example of style binding. Here we can use this technique to bind a color style with an h1 element. The result would be the text with the tag h1 will be displayed in blue color.
1<h1 [style.color]=”blue”>This is a Blue Heading</h1>
From View to Component
Unlike for Component to View, there is only one method to use for data exchange in View to Component and it is known as the event binding technique. For example, there is an event on the left side, let’s say – click. And on the right side, we have a template that consists of methods (myFunction() in this case) and properties that can be bound to the event.
1<button (click)=”myFunction()”>Show alert</button>
In the code above, when the user clicks on the button, the myFunction() method in the component will be called.
Filename app.component.ts
1import { Component } from “@angular/core”;
2 @Component({
3 selector: ‘app-example’,
4 template: `<button (click)=’myFunction()’ >Show alert</button>`
5 })
6 export class AppComponent {
7 myFunction(): void {
8 alert(‘Show alert!’);
9 }
10}
Running the above code will show you the button called “Show Alert”. Clicking the button will bring the myFunction() method into the component to execute the alert() method. As a result, an Alert box will be displayed on the screen with the text “Show an alert”.
Two-way Data Binding in Angular
The most unique offerings of the Angular development framework include two-way data binding. It helps developers to enable bi-directional communication flow to exchange data from view to component and vice versa.
To successfully implement the two data binding features, you need one more thing that comes with Angular and that is the ngModel directive. You can use the syntax below for it is a simple combination of parentheses of event binding and the property binding’s square brackets.
1<input type=”text” [(ngModel)] = ‘val’ />
One other condition you need to fulfill before you can establish two-way data binding is that before using the ngModel directive you must first import the FormsModule from @angular/forms in the app.module.ts file. Because the ngModule directive is stored in the FormsModlule.
Filename app.module.ts
1import { NgModule } from ‘@angular/core’;
2import { BrowserModule } from ‘@angular/platform-browser’;
3import { FormsModule } from “@angular/forms”;
4 import { AppComponent } from ‘./app.component’;
5import { FormsModule } from “@angular/forms”;
6 @NgModule({
7 imports: [BrowserModule, FormsModule],
8 declarations: [ AppComponent],
9 bootstrap: [AppComponent]
10})
11export class AppModule { }
If you directly try to establish the two-way binding without importing the FormsModule first, then you will be left with a Template parse error that will show – “Can’t bind to ‘ngModel’ since it is not a known property of ‘input'”.
But once you have imported the FormModule then you can charge straight up to use ngModule;
1import { Component } from ‘@angular/core’;
2 @Component({
3 selector: ‘app-example’,
4 template: `
5 Enter the value : <input [(ngModel)] =’val’>
6 <br>
7 Entered value is: {{val}}
8 `
9})
10export class AppComponent {
11 val: string = ”;
12}
As a result, you can see an input box on the screen asking for you to insert some value in the View. Now, any value you insert is bound to be displayed as an entered value. For example, if you enter the text – John, it is bound to show the following text – “Entered value is: John”.
Conclusion
In this guide, we discussed the practical implementations of two types of data binding that come with the Angular development framework. Of course, the one-way data binding feature is common among other frameworks but it’s the two-way data binding technique that holds more importance among the community of Angular developers.
Either way, I hope this article can help you implement Angular data binding features. Thanks for reading!