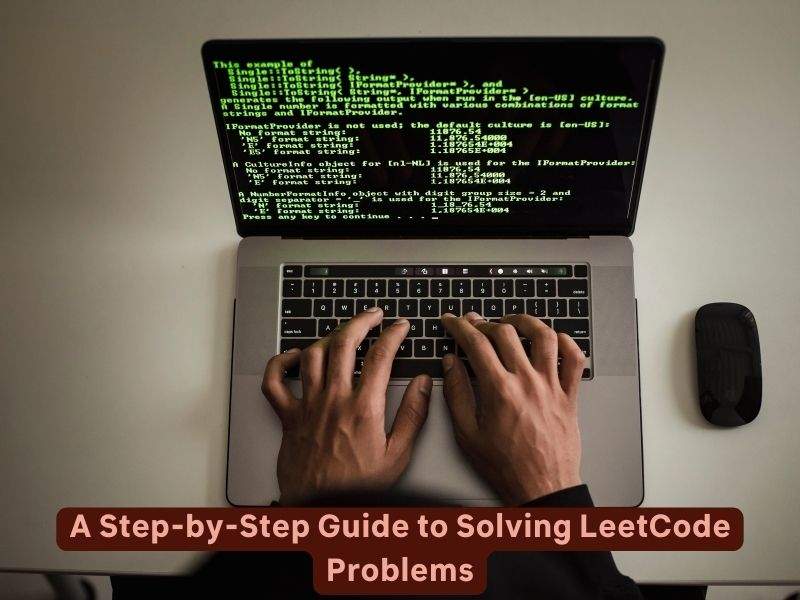
A Step-by-Step Guide to Solving LeetCode Problems
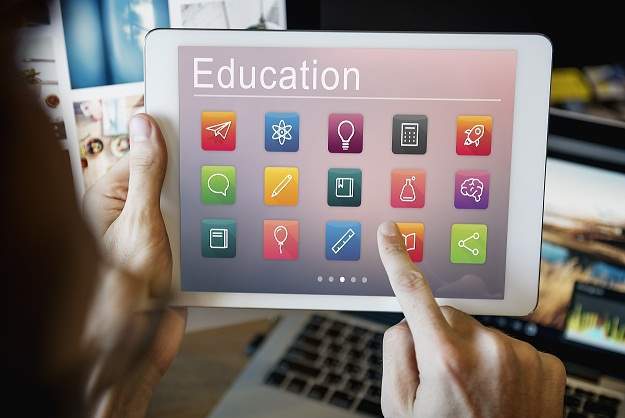
Introduction to Problem-Solving Strategies
We’ll explore the necessary steps and best practices to help you understand, evaluate, and solve LeetCode problems with ease. This involves studying the problem statement and identifying the desired outcome. It’s important to break down complex problems into smaller pieces so they are more manageable. By breaking it down, you can think about each piece independently before tackling the entire problem.
Once you have an understanding of the problem, it’s time to generate ideas. Brainstorming is a great way to come up with potential solutions. Write out all of your ideas, even if they don’t seem likely solutions at first—you may find that thinking through them leads to something workable. It’s best practice here not to get stuck on any single idea keep brainstorming until you have several possible options available.
After generating ideas for possible solutions, it’s time to critically evaluate each one and identify which is most likely to work. Evaluate each solution based on its feasibility, cost efficiency, effectiveness, and any other factors relevant to your particular problem. Use a pros/cons chart or model comparison to objectively compare each option so that you can make an informed decision on which one is best suited for your needs.
Selecting the Right Questions
Selecting the right questions when solving LeetCode problems can be overwhelming. With so many different options and varying levels of difficulty, it can be difficult to know where to start. In this blog post, we’ll provide a step-by-step guide to help you select the best questions and increase your chances for success on LeetCode.
First and foremost, it’s important to understand the problem statement. Take time to read through it carefully and break it down into manageable chunks. Think about the data structures or algorithms that are relevant for solving the problem as well as any other topics or ideas that may help understand the solution.
Once you have an idea of what you need to do, create test cases and edge cases that will allow you to evaluate your progress as you work through the problem. Then, come up with a systematic approach that will help you reach a solution more efficiently. If needed, research online for potential hints or solutions that might help you along the way.
Using Pseudocode & Algorithms
If you’re trying to improve your coding skills, mastering the use of algorithms and pseudocode is essential. But knowing the basics isn’t always enough. To help you understand how to best tackle problems on LeetCode, let’s go through a step-by-step guide to solving LeetCode problems using algorithms and pseudocode.
The first step in problem-solving is understanding the problem. You need to accurately identify what it is asking of you and break it down into elements that can be solved with an algorithm. Once you have a clear understanding of the problem, you are ready to move on to designing a solution using an algorithm. This involves thinking about possible approaches and considering which data structures would be most appropriate for the given task.
After selecting an algorithm approach, it’s time to start writing pseudocode. Pseudocode is a helpful tool when breaking down complex problems and creating rough drafts before implementing actual code. It also allows you to plan for debugging strategies which will be important for when you begin coding your solution.
Now that your pseudocode is written, it’s time to begin coding your solution! As you type out your code, be sure to test for any potential bugs or errors that could occur in the process. Debugging strategies such as adding debugging messages or stepping through each line of code can help you identify any points of concern within your solution.
Writing Code in an Optimal Way
Coding is a complex activity, and writing code optimally requires a keen eye and an analytical approach. But with the right set of strategies and principles, anyone can write effective code to succeed in their programming efforts. Here’s a step-by-step guide to help you optimally write code:
1. Identifying & Analyzing Problems Every coding project starts by identifying the problem that needs to be solved. You should take the time to break down the problem into smaller pieces and analyze it from different angles. You should also ask yourself questions on how your output could work better if there were certain scenarios involved.
2. Understanding Algorithms & Syntax After you’ve identified the problem, it’s important to understand the algorithms and syntax associated with the language of choice for your project. By learning how these work together, you’ll be able to write code more efficiently and accurately solve problems.
3. Proficiency in Language of Choice Once you have a strong grasp of algorithms and syntax, you can start writing code in your language of choice with greater proficiency. Knowledgeable developers often like to use LeetCode problems as practice as they teach essential coding techniques such as recursion, backtracking, dynamic programming, etc., which are useful when faced with complex coding problems.
4. Use of Debugging Tools is essential to identify mistakes or gaps in your code before you submit them for review or testing purposes. Having these tools will allow you to stay on track while adjusting your approach when met with errors or issues while coding along the way so that any changes made are done correctly with minimal effort required for debugging later on down the line. Check Out:-Analytics Jobs
Implementing Optimized Solutions
LeetCode challenges are becoming increasingly popular among developers looking to test their problem-solving capabilities. However, knowing the steps it takes to solve a problem can be daunting. That’s why we’ve created this step-by-step guide on how to successfully implement optimized solutions when tackling LeetCode challenges.
The first step is analyzing the problem. Be sure to become familiar with the prompt and what it is asking you to do. You should also consider any special parameters that may be specified in the challenge by using pseudocode which is a written representation of an algorithm as well as creating diagrams or flowcharts that illustrate the various stages of your solution.
The next stage involves adopting an algorithmic approach to arrive at a solution, making sure you take into account all design considerations that arise from the challenge specifications. Once you’ve come up with the logic for solving the problem, pseudocode construction will help you express this logic in a few concise lines of code. This provides you with an efficient roadmap for completing the challenge and allows you to quickly test its correctness while ironing out any bugs along the way.
After constructing an optimized solution, it’s important to evaluate its performance in terms of time complexity and space complexity. This will give you an indication of how well your solution scales when presented with large amounts of data and helps determine which sections can be improved upon for better overall performance. One strategy for debugging your code efficiently is using breakpoints, as they allow you to inspect parts of your program at runtime and pinpoint errors quickly and reliably.
Debugging & Troubleshooting Strategies
“Debugging and troubleshooting is an integral part of coding. As a software engineer, you will often find yourself stuck in a particular situation while solving a problem. It could be an issue with the logic or incorrect input and output values. Debugging and troubleshooting strategies can help you analyze the problem accurately and efficiently, allowing you to move past the obstacle quickly.
1. Analyze Problem: The first step is to analyze the problem thoroughly by breaking down its structure into smaller components, understanding the approach you need to take, and identifying potential stumbling blocks/challenges within it. This initial analysis will help you save time when trying to solve the problem as it gives a general idea of what needs to be done.
2. Break Down Structure: Once you have broken down the structure of the problem, start thinking through each component in detail, paying close attention to its expected input & output values as well as any special conditions that may affect your approach or implementation of the solution. This breakdown will help give clarity into how each piece interacts with one another for a successful solution.
3. Utilize Resources: For any coding-related problems, there are always resources available online in the form of tutorials or documentation that explain concepts more thoroughly or provide sample codes and solutions for similar problems that you can use as references for your work. Additionally, community forums like Reddit can be a great place for discussing ideas with peers who may better understand underlying issues than you.
Submitting Answers for Review & Feedback
Submitting answers for review and feedback can help you become a better programmer. With LeetCode, you can get instant feedback on your submissions. This guide will walk you through the basics of submitting your code to LeetCode, analyzing mistakes, improving solutions, testing code speed, and optimizing algorithms.
When solving LeetCode problems, it is important to understand the problem statement before attempting to write any code. Once you are familiar with the problem statement, begin writing out your strategy and pseudocode in plain English. This will help you break down a complex problem into manageable steps and prepare you for writing actual code.
Once you have written up your solution and have tested it using sample input/output data cases from LeetCode’s website, submit it for review. When reviewing your solution, make sure that it adheres to the minimum requirements of the problem statement provided by LeetCode. If any errors are found in your submission, try to identify them and make adjustments until all errors have been resolved.
After submitting your solution for review on LeetCode, analyze any mistakes that were present in your original submission. This process involves closely scrutinizing all sections of code and pinpointing opportunities for improvement or optimization. Identifying patterns in the mistakes made is key here; if done correctly this will help prevent those same mistakes from happening again in future solutions.
Utilizing Effective Problem-Solving Strategies
Have you ever found yourself stuck trying to complete a challenging coding problem? If so, you’re not alone. Many coders find themselves overwhelmed by the complexity of some LeetCode problems. Thankfully, with the right strategy in place, these types of problems can be solved quickly and efficiently.
In this blog, we’ll give you a step-by-step guide to solving LeetCode problems effectively. By following these steps, you’ll be able to take on any problem with confidence.
The first step in effectively solving any coding problem is to identify it properly. You should take the time to read through the problem carefully and understand exactly what it’s asking for; don’t rush into writing a solution before you have a firm grasp on what the problem is.
Once you’ve identified the problem, it’s time to analyze it more closely and break it down into smaller parts. This involves looking at how different elements of the problem interact with each other and considering which approaches might work best for solving it.
The next step is to gather resources that can help you tackle the issue more easily. This could involve anything from reading up on algorithms related to your problem to consulting developers who may have faced similar issues in the past. Make sure that whatever resources you use are reliable and up-to-date.